This merge includes updated comments in the ElasticVectorSearch class to
provide information on how to connect to `Elasticsearch` instances that
require login credentials, including Elastic Cloud, without any
functional changes.
The `ElasticVectorSearch` class now inherits from the `ABC` abstract
base class, which does not break or change any functionality. This
allows for easy subclassing and creation of custom implementations in
the future or for any users, especially for me 😄
I confirm that before pushing these changes, I ran:
```bash
make format && make lint
```
To ensure that the new documentation is rendered correctly I ran
```bash
make docs_build
```
To ensure that the new documentation has no broken links, I ran a check
```bash
make docs_linkcheck
```
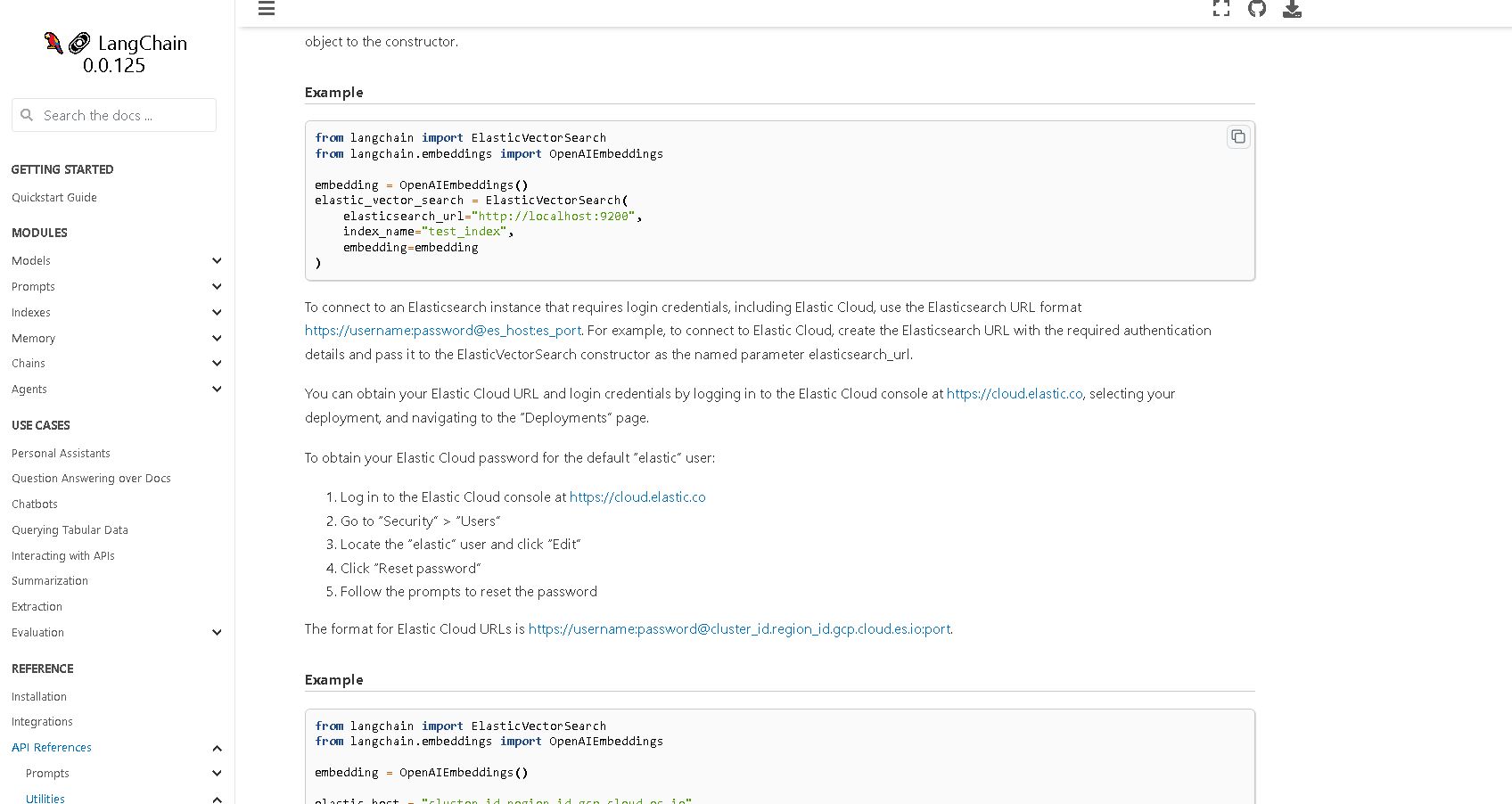
Also take a look at https://github.com/hwchase17/langchain/issues/1865
P.S. Sorry for spamming you with force-pushes. In the future, I will be
smarter.