mirror of https://github.com/hwchase17/langchain
wfh/happyfamily
eugene/memory_equivalents_simplify
master
v0.2
cc/pdf_docs
eugene/qa_test_2
eugene/ci_fix_question_template
erick/partners-box-release-0-2-2
eugene/add_memory_equivalents
isaac/toolerrorpassing
wfh/toks
bagatur/enable_azure_feats
cc/extended_tests
v0.1
cc/release_mongo
eugene/robocorp
eugene/foo
eugene/pydantic_v1_foo
erick/community-release-0-2-17
erick/docs-mdx-v3-compat-wip
eugene/update_is_caller_internal
isaac/toolerrorhandling03
bagatur/des_from_path
bagatur/v0.3/preview_api_ref
bagatur/run_tutorials
bagatur/run_all_docs_how_to
isaac/toolerrorhandling
bagatur/rfc_docs_gha
eugene/foo_meow
bagatur/fix_embeddings_filter_init
wfh/keyword_runnable_like_bu
eugene/add_mypy_plugin
eugene/v0.3_wut
bagatur/core_0_3_0_dev1
bagatur/v0.3rc_merge_master_2
bagatur/v0.3rc_merge_master
eugene/core_0.3rc_first
bagatur/oai_emb_fix
isaac-recursiveurlloader-testing
harrison/3.0
bagatur/core_pydocstyle_lint
harrison/support-kwargs-vectorstore
wfh/more_interops
bagatur/format_content_as
erick/community-undo-azure-ad-access-token-breaking-change
isaac/responseformatstuff
bagatur/delight
erick/ai21-integration-test-fixes
erick/all-more-lint-additions
erick/infra-continue-on-error
erick/ai21-address-breaking-changes-in-sdk-2-14-0-wip
bagatur/rfc_anthropic_cache_usage
bagatur/json_mode_standard
bagatur/dict_msg_tmpl2
wfh/nowarn
bagatur/rfc_dont_update_run_name
bagatur/oai_disabled_params
jacob/templates
bagatur/content_block_template
erick/chroma-fix-typing
isaac/moreembeddingtests
eugene/openai_0.3
bagatur/standard_tests_async
eugene/merge_pydantic_3_changes
eugene/0.3_release_docs
cc/deprecate_evaluators
bagatur/selector_add_examples
bagatutr/langsmith_example_selector
docs/fix_chat_model_os
eugene/security_related
eugene/add_async_api
eugene/integration_docs_cohere
eugene/multimodal_embedding_model
isaac/moretooltables
bagatur/merged_docs_styling
eugene/clean_up_pre_init
eugene/v0.3_meow
bagatur/serialize-pydantic-metadata
eugene/fix_tool_extra
erick/infra-pydantic-v2-scheduled-testing
bagatur/add_pydantic_sys_info
eugene/community_draft
cc/fix_exp_ci
eugene/pydantic_v_3
erick/docs-llm-embed-index-tables-wip
eugene/update_llm_result_types
eugene/add_json_schema_methods
bagatur/docs_versioning
bagatur/simplify_docs_header_footer
bagatur/docs_cp_ls_theme
erick/cli-new-template-types
isaac/ollamauniversalchat
isaac/llmintegrationtests
bagatur/fewshot_scratch
isaac/ollamallmfix
wfh/warn_name
eugene/add_indexer_to_retriever
bagatur/07_26_24/poetry_lock
cc/toolkits
erick/docs-new-integrations-docs
cc/api_chain
wfh/link
eugene/rate_limiting_requests
eugene/add_rate_limiter_integrations
isaac/ollamaimageissues
cc/many_tools_guide
eugene/update_relative_imports
eugene/add_timeout_for_tests
isaac/tavilynewlinefix
isaac/create_react_agent_doc_fix
eugene/add_tests_for_pydantic_models
eugene/document_indexer_v2
bagatur/ruff_0_5_3
wfh/parent
wfh/async_chromium
erick/infra-try-removing-uv-for-editable-install
eugene/indexing_abstraction_minimal
jacob/currying_docs
jacob/curry_tools
eugene/indexing_abstraction
wfh/triggered
cc/bind_tools
jacob/docs_style
isaac/fewshotpromptdocs
eugene/migrate_graphvectorstore_to_community
jacob/tools_additional_params
wfh/curry
wfh/inherit
eugene/stores_new
bagatur/rfc_tool_call_id_in_config
bagatur/docs_intro_wording
bagatur/lint_all_core_deps
eugene/update_add_texts
eugene/index_manager
eugene/tracing_interop2
eugene/migrate_vectorstores
eugene/cleanup_deprecated_code
wfh/is_error
eugene/root_validators_02
eugene/root_validators_03
eugene/pinecone_add_standard_tests
eugene/indexing_v2
wfh/url
bagatur/rfc_configurable_model
cc/tool_token_counts
renderer
bagatur/mypy_v1_update
wfh/add_list_support
bagatur/rfc_tool_call_filter
erick/anthropic-release-0-1-16
wfh/add_tool_param_descripts_2
wfh/add_tool_param_descripts
nc/19jun/core-no-pydantic
bagatur/opinionated_formatter
bagatur/rfc_docstring_lint
cc/fix_milvus
cc/update_pydantic_dep
maddy/support-options-in-langchainhub
isaac/chatopenaiparalleltoolcallingparam
erick/huggingface-relax-tokenizers-dep
rlm/test-llama-cpp
bagatur/retrieval_v2_scratch
erick/core-loosen-packaging-lib-version
eugene/disable_lint_rule
eugene/get_model_defaults
wfh/allyourtreesarebelongtome
eugene/pydantic_migration_2_b
isaac/sitemaploader-goldendocs
bagatur/recursive_url_bash
erick/docs-update-chatbedrock-with-tool-calling-docs-dont-use
erick/docs-update-chatbedrock-with-tool-calling-docs-do-not-use
isaac/sitemaploader-testing
isaac/sitemaploader-tests
eugene/pydantic_migration_2
erick/core-throw-error-on-invalid-alternative-import-in-deprecated
erick/core-throw-errors-on-invalid-alternative-import
bagatur/rfc_smithify_docs
eugene/async_history_2
bagatur/ai21_0_1_6
erick/docs-rewrite-contributor-docs
cc/update_openai_streaming_token_counts
eugene/llm_token_counts
eugene/langchain_how_to_config
bagatur/docs-format-api-ref
eugene/callbacks_propagate
erick/docs-v02-url-rfc
maddy/default-prompt-private-in-hub
eugene/update_version_docs
bagatur/parse_tool_docstring_fix
erick/docs-algolia-api-key-update
eugene/how_does_this_stream
eugene/langchain_core_manager
eugene/update_linting
erick/docs-ignore-echo-false-blocks
dqbd/api_ref_styles
erick/infra-codespell-v1
erick/infra-codespell-in-v1-branch
erick/community-release-0-2-0rc1
eugene/add_change_log2
harrison/new-docs
cc/retriever_score
bagatur/community_0_0_37
wfh/may3/help
eugene/core_0.2.0rc1
cc/docs_build
eugene/update_warnings2
bagatur/oai_tool_choice_required
cc/fix_openai
wfh/add_rid_to_chain
eugene/migrate_document_loaders
bagatur/mistral_client
wfh/add_parameter_descriptions
erick/core-remove-batch-size-from-llm-start-callbacks
eugene/refactor_deprecations
eugene/release_0_2_0
eugene/web_retriever
eugene/move_memories_2
bagatur/tryout_uv
eugene/entity_store
eugene/run_type_for_lambdas
bagatur/rfc_standardize_input_msgs
bagatur/rfc_serialized_tool
brace/show-last-update-docs
erick/release-note-experiments
eugene/runnable_config
cc/function_message
rlm/rag_eval_guide
bagatur/rfc_token_usage
eugene/custom_embeddings
eugene/community_fix_imports
bagatur/goog_doc_nit
erick/docs-runnable-list-operations
bagatur/rm_convert_to_tool_docs
eugen/providers_update
erick/core-deprecate-vectorstore-relevance-scoring
eugene/outline_wrapper_1
erick/pytest-experiments-2
erick/pytest-experiments
erick/partner-cloudflare
rlm/langsmith_testing
erick/community-patch-clickhouse-make-it-possible-to-not-specify-index
eugene/postgres_vectorstore
bagatur/openllm_new_api
bagatur/layerupai
cc/deprecated_imports
erick/cohere-adaptive-rag-cookbook
erick/cohere-multi-tool-integration-test
dqbd/openai-lax-jsonschema
eugene/xml_again
brace/format-dpcs
eugene/pull_to_funcs
bagatur/fix_getattr
erick/core-patch-placeholder-message-shorthand
bagatur/0.2
eugene/unsafe_pydantic
bagatur/community_migration_script
bagatur/versioned_docs_2
bagatur/versioned_docs
bagatur/find_broken_links
bagatur/stream_pydantic
wfh/add_hub_version
eugene/stackframe
wfh/log_error
wfh/add_eval_metadata
erick/airbyte-patch-baseloader-wip
bagatur/rename_msg_kwargs
wfh/specify_version
fork/feature_audio_loader_auzre_speech
erick/infra-remove-venv-from-poetry-cache
erick/ci-test-timeout
erick/test-community-ci
erick/test-ci
wfh/add_warnings
eugene/huggingface
erick/core-patch-community-patch-baseloader-to-core
erick/core-minor-multimodal-document-page-content-rfc
bagatur/support_pydantic_context
bagatur/rfc_structured_list
erick/test-partner-failure
erick/test-partner-success
erick/test-error
eugene/fix_openai_community_stream
erick/test-ci-should-fail
erick/testutils
jacob/people
erick/docs-remove-platforms-redirect
eugene/add_people
erick/infra-check-min-versions-in-pr-ci
langchain-ai/langchain@5cbabbd
eugene/test_lint
erick/exa-lint
bagatur/make_cohere_client_optional
bagatur/rfc_as_str
erick/infra--individual-template-ci-
bagatur/rfc_@chain_typing
erick/infra-try-1-job-sphinx-build
rlm/mistral_cookbook
bagatur/rfc_chat_invoke_llm_res
erick/infra-rtd-build-bump-null
erick/infra-rtd-build-bump
erick/autoapi-test
bagatur/speedup_sphinx
erick/community-lint
erick/partner-nomic
erick/cli-langchain-dep-versions
bagatur/init_chat_prompt_msg_like
wfh/custom_prompt
fork/async-doc-loader
rlm/rag_from_scratch
eugene/message_history_test
erick/api-ref-navbar-update
bagatur/rfc_bind_collision
bagatur/bind_outside_agent
erick/release-notes
jacob/chatbot_message_passing
bagatur/3_12_ci
bagatur/assign_unpack
bagatur/docs_top_nav
bagatur/batch_overload_typing
bagatur/core_0_1_15_rc_1
bagatur/rfc_rich_retrieval
bagatur/runnable_drop
bagatur/class_chain
bagatur/initial_tool_choices
eugene/streaming_events
erick/core-patch-fallbacks-error-chain
harrison/tool-invocation
bagatur/tool_executor
erick/deepinfra-chat
eugene/agents_docs
eugene/update_index.md
bagatur/rfc_tool_executor
bagatur/rfc_extraction_improvement
bagatur/downgrade_setup_python
erick/mistralai-patch-enforce-stop-tokens
bagatur/thread_inof
bagatur/docs_last_updated_2
bagatur/docs_last_updated
erick/google-docs
dqbd/json-output-oai-parser-serialization
bagatur/cli_pkg_tmpl_lc_ver
erick/infra-try-show-last-update-time
bagatur/try_stat
bagatur/core_tracer_backwards_compat
erick/infra-ci-python-matrix-update-3-12
bagatur/rfc_retriever_return_str
do-not-merge
bagatur/dispatch_main_ci
bagatur/show_last_update_time
harrison/docs-m
harrison/docs-revamp-mirror
harrison/new-docs-revamp
harrison/agents-rewrite-code
bagatur/api_flyout
harrison/revamp-memory
harrison/merged-branches
bagatur/stuff_docs_lcel
harrison/agent-docs-concepts
harrison/agent-docs-custom
bagatur/api-ref-navbar-update
bagatur/combine_docs_chain_as_runnable
erick/ci-test-do-not-merge
bagatur/chat_hf
erick/infra--run-ci-on-all-prs-
bagatur/core_update_ruff_mypy
erick/nbconvert
bagatur/lc_stack_update
wfh/bind_tools
eugene/fix_xml_agent
harrison/anthropic-package
wfh/vertexai_fixup
bagatur/core_0_0_13
wfh/add_oai_agent_core_examples
erick/docs-bullet-points
wfh/gemini
eugene/bug_history
bagatur/community
harrison/turn-off-serializable
harrison/serializable-baga
wfh/prevent_outside
harrison/mongo-agent
erick/all-patch---change-ci-title-in-event-of-no-matrix-expansion-
rlm/update-img-prompt
eugene/update_file_chat_memory
harrison/deepsparse
bagatur/core_0.1
harrison/integrations
erick/docs-docusaurus-3
rlm/mm-rag-deck
bagatur/core_lint_docstring
bagatur/core_0_0_8
bagatur/lcel_get_started
bagatur/fmt_notebooks
bagatur/export_prompt_chat_classes
harrison/add-imports
bagatur/serialization_tests
bagatur/patch_0.0.400
wfh/tqdm_for_wait
bagatur/fix_core_namespace
erick/core-namespace-same
erick/api-docs-core-bugfix-
brace/new-lc-stack-svg
wfh/tqdm_wait
v0.0.339
bagatur/cogniswitch
dqbd/docs-responsivity-fix
bagatur/multi_return_source
wfh/func_eval
bagatur/full_template_docs
bagatur/callbacks-refactor
(vectorstore)/PGVectorAsync
rlm/mm_template
bagatur/rfc_bind_getattr
erick/skip-release-check-cli
bagatur/rm_return_direct_error
rlm/sql-pgvector-template
erick/improvement-format-notebooks
erick/improvement-default-docs-url-root
bagautr/rfc_image_template
refactorChromaInitLogic
rlm/ollama_json
bagatur/rfc_pinecone_hybrid
eugene/document_runnables2
harrison/root-listeners
wfh/add_llm_output_to_adapter
rlm/biomedical-rag
bagatur/cohere_input_type
bagatur/update-schema
erick/cli-codegen
wfh/content_union
bagatur/docs_smith_serve
rlm/open_clip_embd_expt
rlm/multi-modal-template
wfh/ossinvoc
pg/test-publish-rc-versions
wfh/conversational_feedback
bagatur/voyage-ai
template-readme-missing-env
rescana-com/master
bagatur/lakefs-loader
bagatur/readthedocs-loader-improvements
hwchase17-patch-1
eugene/fix_type_onbase_transformer
bagatur/deep_memory_version_1
api-reference-agents-functions
erick/cli-ci
bagatur/retry_nit
wfh/tree_distance
jacoblee93-patch-1-1
wfh/runnable_traceable
bagatur/rfc_chat_batch_gen
rlm/text-to-pgvector
bagatur/e2b-integration2
bagatur/api-reference-agents-functions
shorthills-ai/master
bagatur/e2b-integration
wfh/save_model_name
bagatur/voyage
rlm/LLaMA2_sql_scrub
bagatur/cogniswitch_chains
bagatur/private_fn
erick/langservehub
bagatur/rfc_vecstore_interface
nc/repl-lib
charlie/fine-tuning-notebook
harrison/move-imports
wfh/rtds
wfh/json_schema_evaluator
pg/python-3.12
wfh/eval_public_dataset
ankush/single-generations
nc/pandas-eval
eugene/update_warning_class
ankush/single-input
ankush/delete_v1_tracer
wfh/background
bagatur/bump_304
bagatur/dedup_transformer
eugene/fix_webbase_loader
harrison/move-pydantic-v1
wfh/vectorstore_tracing
vdaas-feature/vald
harrison/agents-exoskelton-1
harrison/agents-exoskeleton
jacob/routing_cookbook
harrison/more-imports
harrison/remove-from-init
eugene/automaton_variant_4
harrison/specified-input-keys
bagatur/docs_zoom
jacob/feature_vercel_analytics
wfh/update_types
francisco/sql_agent_improvements
wfh/implicit_client
bagatur/auto_rewrite_retrieval
bagatur/konko
eugene/automaton_variant_3
wfh/default_retries
bagatur/lint_fix
rlm/fix-prompts
wfh/redirects
eugene/automaton_variant_2
bagatur/fix_multiquery
wfh/json_other
wfh/fix_link
bagatur/add-data-anonymizer
bagatur/mem_session
molly/vectorstore-batching
deepsense-ai/llama-cpp-grammar
bagatur/gpt_4_docstring
harrison/add-llm-kwargs
bagatur/redis_refactor
rlm/llama-grammar
bagatur/runnable_mem
wfh/clirun
eugene/document_pipeline
harrison/retrieval-agents
bagatur/rfc_fallback_inherit
bagatur/epsilla
bagatur/promptguard
harrison/pydantic-bridge
bagatur/cheatsheet
wfh/update_criteria_prompt
pydantic/b2_bump
eugene/pydantic_v2_tools2
wfh/criteria_strat
eugene/wrap_openapi_stuff
bagatur/bump_264
bagatur/new_msg
rlm/agent_use_case
harrison/remove-things-from-init
harrison/clean-up-imports
bagatur/lite_llm
bagatur/rfc_zep_search
bagatur/pydantic_agnostic
bagatur/bagel
bagatur/fix_sched_2
wfh/async_eval_default
bagatur/respect_light_mode
bagatur/docsly
eugene/automaton_variant_1
wfh/return_exceptions
wfh/example_id_config
bagatur/rm_nuclia_ext
wfh/fix_recursive_url_loader
bagatur/runnable_locals
wfh/embeddings_callbacks_v3
bagatur/google_drive
rlm/chatbots_use_case
wfh/langsmith_nopydantic
eugene/enum_rendering
harrison/add-memory-to-sql
bagatur/rfc_fallbacks
harrison/xml-agent
bagatur/mod_desc
wfh/memory_interface
wfh/throw_on_broken_links
eugene/expand_documentation
wfh/api_ref
wfh/swizzle
eugene/test
harrison/async-web
harrison/fix-typo
wfh/retriever_additional_data
harrison/experimental-package
bagatu/rfc_pkg_per_chain
wfh/default_data_type
harrison/move-to-schema/chain
harrison/move-to-schema-more-callbacks
wfh/to_prompt_template
wfh/not_implemented
wfh/limit_concurrency
wfh/delete_deprecated
harrison/move_to_core
harrison/move-to-core/prompts
wfh/add_agent_trajectory_loader
ankush/message-eval
harrison/variable-table
wfh/skip_no_output
harrison/apply-async
harrison/improve-docs-formatting
vwp/embedding_fuzzy
wfh/evals_docs_reorg_draft
vwp/comparison_with_references
wfh/comparison_with_references
harrison/split-schema-dir
vwp/accept_no_reasoning
wfh/embeddings_callbcaks
vwp/fix_promptlayer
wfh/key_matching
harrison/marqo
vwp/make_new_eval_chain_run
vwp/any_callable
vwp/time_to_first_token
vwp/accept_chain
vwp/evals_docs_reorg
vwp/similarity
vwp/use_langsmith
vwp/rm_dep
vwp/script_for_adding_docs
octoml/master
harrison/set-pydantic-docs
harrison/markdown-docs
vwp/drafts/unit_testing
harrison/functions
ankush/asyncio-gather-agenerate
vwp/retriever_callbacks_v2
vwp/schema_dir
harrison/allow-kwargs
eugene/persistence_db
vwp/anthropic_token_usage
vwp/evaluator_chains
vwp/envurl
eugene/research_v1
eugene/chain_generics
harrison/neo4j-lint
ankush/callbacks-cleanup
dev2049/pgvector_fix
harrison/anthropic-chat
vwp/simplify_tracer2
vwp/simplify_tracer
harrison/schema-directory
harrison/comp-prompt
dev2049/rough_draft_doc_manager
vwp/child_runs
ankush/chat-agent-parsing
dev2049/combine_quickstart
dev2049/concise_get_started
vwp/feedback_crud
harrison/exclude-embedings
dev2049/azure_vecstore
vwp/base_model
dev2049/getting_started_clean
dev2049/change_llm_name
dev2049/embedding_rename
eugene/prompt_template
harrison/serialize-chat
dev2049/embed_docs_to_texts
dev2049/doc_clean
dev2049/chroma_cleanup
harrison/few-shot-w-template-fix
retrievalqafinetune
dev2049/retrieval_eval
vwp/tracing_docs
vwp/bold_headergs
eugene/add_file_system
harrison/return-prompt
vwp/tracer-async-call
eugene/check_something
dev2049/combine_refac
eugene/updat_extended_tests
eugene/meow_draft
eugene/fix_google_palm_tests
vwp/dcv2
eugene/retriever_version
tjaffri/dgloader
harrison/pdfplumber
vwp/patch
harrison/character-chat-agent
harrison/mongo-loader
harrison/sharepoint
eugene/add_caching_from_master_only
dev2049/save-to-notion-tool
dev2049/self_query_integration
dev2049/update_lock
eugene/revert_workflows
revert-4465-harrison/env-var
dev2049/pgvector-size-fix
vwp/eval_examples
fork-chains
eugene/test_branch
vwp/add-github-api-utility
vwp/from_llm_and_tools
vwp/pandas_cb_manager
add-scenexplain-tool
vwp/tools_callbacks
vwp/relax_chat_agent
vwp/parser__type
vwp/filter_ambiguous_args
harrison/get-working-with-agents
dev2049/null_callback_hack
dev2049/llm_requests_chain
vwp/test_on_built_wheel
vwp/avoid_poetry_deps_in_ci
eugene/openai_optional
vwp/agent_tests
vwp/structured_tools
vwp/align_search_tools
vwp/structured_tools_with_pyd
vwp/inheritance_same_agents
vwp/chatregtests
dev2049/default_models
dev2049/perfect_retriever
dev2049/docs_stateful
vwp/add_args
khimaros/master
vwp/chroma_elements
vwp/default_dont_raise
vwp/lintfix
harrison/anthropic
dev2049/retrieval_eval_nb
harrison/contextual-compression
vwp/marathon
agents-4-18
harrison-outerr-exc
vwp/hf_image_gen
vwp/hf_imagen
vwp/tools_undo
vwp/characters_2
vwp/tools-refactor-2
harrison/autogpt
harrison/typeo
dev2049/fmt_nbs
vwp/numexpr
harrison/characters-nb
vwp/characters_with_planning
harrison/pinecone-backwards-compat
vwp/openapi_with_tool_retrieval
harrison/aws-text
ankush/patch1
harrison/processor
harrison/script-update
harrison/api-chain
harrison/ai21-embeddings
harrison/alpaca
nc/poe-handler-chat-model
nc/poe-handler
harrison/mrkl-parser
harrison/agent-experiments
harrison/replicate
harrison/chat-chain
harrison/update-wandb
harrison/debug
harrison/qasper
harrison/dbpedia
harrison/changes
jeremy/guardrails
nc/guardrails-error-handling
harrison/guardrails
harrison/use-output-parsers
John-Church-guard
agent_evaluation
harrison/kor-chain
harrison/inference-api
ankush/callback-refactor
harrison/eval
harrison/audio
ankush/prompt-abstractions
harrison/memory-chat
harrison/indexes
ankush/partial-prompt-apply
harrison/sagemaker
harrison/datetime
harrison/openapiagent
harrison/paged-pdf
harrison/pswsl
ankush/example-runner
harrison/guards
scad/api-chain
harrison/prompt-bugs
harrison/sql-agent
harrison/pinecone-try-except
harrison/callback-updates
harrison/map-rerank
harrison/combine-docs-parse
harrison/azure-rfc
harrison/sequential_chain_from_prompts
harrison/agent-refactor
harrison/agent_intermediate_steps
harrison/agent_multi_inputs
harrison/promot-mrkl
harrison/fix_logging_api
harrison/use_output_parser
harrison/track_intermediate_steps
harrison/sql_error
harrison/logging_to_file
harrison/output_parser
harrison/flexible_model_args
harrison/agent-improvements
harrison/router_docs
harrison/docs
samantha/add_llm_to_example
harrison/reorg_smart_chains
mako-templates
harrison/save_metadatas
harrison/router
harrison/custom_pipeline
harrison/chain_pipeline
harrison/prompts_docs
harrison/attempt_citing_in_prompt
harrison/load_prompt
harrison/prompts_take_2
harrison/ape
harrison/prompt_examples
harrison/add_dependencies
langchain-ai21==0.1.4
langchain-ai21==0.1.5
langchain-ai21==0.1.6
langchain-ai21==0.1.7
langchain-airbyte==0.1.1
langchain-anthropic==0.1.12
langchain-anthropic==0.1.13
langchain-anthropic==0.1.14rc1
langchain-anthropic==0.1.14rc2
langchain-anthropic==0.1.15
langchain-anthropic==0.1.16
langchain-anthropic==0.1.17
langchain-anthropic==0.1.18
langchain-anthropic==0.1.19
langchain-anthropic==0.1.20
langchain-anthropic==0.1.21
langchain-anthropic==0.1.22
langchain-anthropic==0.1.23
langchain-anthropic==0.2.0
langchain-anthropic==0.2.0.dev0
langchain-anthropic==0.2.0.dev1
langchain-anthropic==0.2.1
langchain-azure-dynamic-sessions==0.1.0
langchain-azure-dynamic-sessions==0.1.0rc0
langchain-azure-dynamic-sessions==0.2.0
langchain-box==0.1.0
langchain-box==0.2.0
langchain-box==0.2.1
langchain-chroma==0.1.1
langchain-chroma==0.1.2
langchain-chroma==0.1.4
langchain-cli==0.0.22
langchain-cli==0.0.23
langchain-cli==0.0.24
langchain-cli==0.0.25
langchain-cli==0.0.26
langchain-cli==0.0.27
langchain-cli==0.0.28
langchain-cli==0.0.29
langchain-cli==0.0.30
langchain-cli==0.0.31
langchain-community==0.0.35
langchain-community==0.0.36
langchain-community==0.0.37
langchain-community==0.0.38
langchain-community==0.2.0
langchain-community==0.2.0rc1
langchain-community==0.2.1
langchain-community==0.2.10
langchain-community==0.2.11
langchain-community==0.2.12
langchain-community==0.2.13
langchain-community==0.2.14
langchain-community==0.2.15
langchain-community==0.2.16
langchain-community==0.2.17
langchain-community==0.2.2
langchain-community==0.2.3
langchain-community==0.2.4
langchain-community==0.2.5
langchain-community==0.2.6
langchain-community==0.2.7
langchain-community==0.2.9
langchain-community==0.3.0
langchain-community==0.3.0.dev1
langchain-community==0.3.0.dev2
langchain-core==0.1.47
langchain-core==0.1.48
langchain-core==0.1.50
langchain-core==0.1.51
langchain-core==0.1.52
langchain-core==0.2.0
langchain-core==0.2.0rc1
langchain-core==0.2.1
langchain-core==0.2.10
langchain-core==0.2.11
langchain-core==0.2.12
langchain-core==0.2.13
langchain-core==0.2.15
langchain-core==0.2.16
langchain-core==0.2.17
langchain-core==0.2.18
langchain-core==0.2.19
langchain-core==0.2.2
langchain-core==0.2.20
langchain-core==0.2.21
langchain-core==0.2.22
langchain-core==0.2.23
langchain-core==0.2.24
langchain-core==0.2.25
langchain-core==0.2.26
langchain-core==0.2.27
langchain-core==0.2.28
langchain-core==0.2.29
langchain-core==0.2.29rc1
langchain-core==0.2.2rc1
langchain-core==0.2.3
langchain-core==0.2.30
langchain-core==0.2.31
langchain-core==0.2.32
langchain-core==0.2.33
langchain-core==0.2.34
langchain-core==0.2.35
langchain-core==0.2.36
langchain-core==0.2.37
langchain-core==0.2.38
langchain-core==0.2.39
langchain-core==0.2.4
langchain-core==0.2.40
langchain-core==0.2.41
langchain-core==0.2.5
langchain-core==0.2.6
langchain-core==0.2.7
langchain-core==0.2.8
langchain-core==0.2.9
langchain-core==0.3.0
langchain-core==0.3.0.dev1
langchain-core==0.3.0.dev2
langchain-core==0.3.0.dev3
langchain-core==0.3.0.dev4
langchain-core==0.3.0.dev5
langchain-core==0.3.1
langchain-core==0.3.2
langchain-couchbase==0.0.1
langchain-couchbase==0.1.0
langchain-couchbase==0.1.1
langchain-exa==0.1.0
langchain-exa==0.2.0
langchain-experimental==0.0.58
langchain-experimental==0.0.59
langchain-experimental==0.0.60
langchain-experimental==0.0.61
langchain-experimental==0.0.62
langchain-experimental==0.0.63
langchain-experimental==0.0.64
langchain-experimental==0.0.65
langchain-experimental==0.3.0
langchain-experimental==0.3.0.dev1
langchain-fireworks==0.1.3
langchain-fireworks==0.1.4
langchain-fireworks==0.1.5
langchain-fireworks==0.1.6
langchain-fireworks==0.1.7
langchain-fireworks==0.2.0
langchain-fireworks==0.2.0.dev0
langchain-fireworks==0.2.0.dev1
langchain-fireworks==0.2.0.dev2
langchain-groq==0.1.10
langchain-groq==0.1.4
langchain-groq==0.1.5
langchain-groq==0.1.6
langchain-groq==0.1.8
langchain-groq==0.1.9
langchain-groq==0.2.0
langchain-groq==0.2.0.dev0
langchain-groq==0.2.0.dev1
langchain-huggingface==0.0.1
langchain-huggingface==0.0.2
langchain-huggingface==0.0.3
langchain-huggingface==0.1.0
langchain-huggingface==0.1.0.dev1
langchain-ibm==0.1.5
langchain-ibm==0.1.6
langchain-ibm==0.1.7
langchain-ibm==0.1.8
langchain-ibm==0.1.9
langchain-milvus==0.1.0
langchain-milvus==0.1.1
langchain-milvus==0.1.2
langchain-milvus==0.1.3
langchain-milvus==0.1.4
langchain-milvus==0.1.5
langchain-mistralai==0.1.10
langchain-mistralai==0.1.11
langchain-mistralai==0.1.12
langchain-mistralai==0.1.13
langchain-mistralai==0.1.6
langchain-mistralai==0.1.7
langchain-mistralai==0.1.8
langchain-mistralai==0.1.9
langchain-mistralai==0.2.0
langchain-mistralai==0.2.0.dev1
langchain-mongodb==0.1.4
langchain-mongodb==0.1.5
langchain-mongodb==0.1.6
langchain-mongodb==0.1.7
langchain-mongodb==0.1.8
langchain-mongodb==0.1.9
langchain-mongodb==0.2.0
langchain-mongodb==0.2.0.dev1
langchain-nomic==0.1.0
langchain-nomic==0.1.1
langchain-nomic==0.1.2
langchain-nomic==0.1.3
langchain-ollama==0.1.0
langchain-ollama==0.1.1
langchain-ollama==0.1.2
langchain-ollama==0.1.3
langchain-ollama==0.2.0
langchain-ollama==0.2.0.dev1
langchain-openai==0.1.10
langchain-openai==0.1.11
langchain-openai==0.1.12
langchain-openai==0.1.13
langchain-openai==0.1.14
langchain-openai==0.1.15
langchain-openai==0.1.16
langchain-openai==0.1.17
langchain-openai==0.1.19
langchain-openai==0.1.20
langchain-openai==0.1.21
langchain-openai==0.1.21rc1
langchain-openai==0.1.21rc2
langchain-openai==0.1.22
langchain-openai==0.1.23
langchain-openai==0.1.24
langchain-openai==0.1.25
langchain-openai==0.1.5
langchain-openai==0.1.6
langchain-openai==0.1.7
langchain-openai==0.1.8
langchain-openai==0.1.8rc1
langchain-openai==0.1.9
langchain-openai==0.2.0
langchain-openai==0.2.0.dev0
langchain-openai==0.2.0.dev1
langchain-openai==0.2.0.dev2
langchain-pinecone==0.1.1
langchain-pinecone==0.1.2
langchain-pinecone==0.1.3
langchain-pinecone==0.2.0
langchain-pinecone==0.2.0.dev1
langchain-prompty==0.0.1
langchain-prompty==0.0.2
langchain-prompty==0.0.3
langchain-prompty==0.1.0
langchain-qdrant==0.0.1
langchain-qdrant==0.1.0
langchain-qdrant==0.1.1
langchain-qdrant==0.1.2
langchain-qdrant==0.1.3
langchain-qdrant==0.1.4
langchain-qdrant==0.2.0.dev1
langchain-robocorp==0.0.10
langchain-robocorp==0.0.10.post1
langchain-robocorp==0.0.6
langchain-robocorp==0.0.7
langchain-robocorp==0.0.8
langchain-robocorp==0.0.9
langchain-robocorp==0.0.9.post1
langchain-text-splitters==0.0.2
langchain-text-splitters==0.2.0
langchain-text-splitters==0.2.1
langchain-text-splitters==0.2.2
langchain-text-splitters==0.2.4
langchain-text-splitters==0.3.0
langchain-text-splitters==0.3.0.dev0
langchain-text-splitters==0.3.0.dev1
langchain-together==0.1.1
langchain-together==0.1.2
langchain-together==0.1.3
langchain-together==0.1.4
langchain-together==0.1.5
langchain-unstructured==0.1.0
langchain-unstructured==0.1.1
langchain-unstructured==0.1.2
langchain-unstructured==0.1.4
langchain-upstage==0.1.4
langchain-upstage==0.1.5
langchain-voyageai==0.1.1
langchain-voyageai==0.1.2
langchain==0.1.17
langchain==0.1.19
langchain==0.1.20
langchain==0.2.0
langchain==0.2.0rc1
langchain==0.2.0rc2
langchain==0.2.1
langchain==0.2.10
langchain==0.2.11
langchain==0.2.12
langchain==0.2.13
langchain==0.2.14
langchain==0.2.15
langchain==0.2.16
langchain==0.2.2
langchain==0.2.3
langchain==0.2.4
langchain==0.2.5
langchain==0.2.6
langchain==0.2.7
langchain==0.2.8
langchain==0.2.9
langchain==0.3.0
langchain==0.3.0.dev1
langchain==0.3.0.dev2
v0.0.1
v0.0.100
v0.0.101
v0.0.102
v0.0.103
v0.0.104
v0.0.105
v0.0.106
v0.0.107
v0.0.108
v0.0.109
v0.0.110
v0.0.111
v0.0.112
v0.0.113
v0.0.114
v0.0.115
v0.0.116
v0.0.117
v0.0.118
v0.0.119
v0.0.120
v0.0.121
v0.0.122
v0.0.123
v0.0.124
v0.0.125
v0.0.126
v0.0.127
v0.0.128
v0.0.129
v0.0.130
v0.0.131
v0.0.132
v0.0.133
v0.0.134
v0.0.135
v0.0.136
v0.0.137
v0.0.138
v0.0.139
v0.0.140
v0.0.141
v0.0.142
v0.0.143
v0.0.144
v0.0.145
v0.0.146
v0.0.147
v0.0.148
v0.0.149
v0.0.150
v0.0.151
v0.0.152
v0.0.153
v0.0.154
v0.0.155
v0.0.156
v0.0.157
v0.0.158
v0.0.159
v0.0.160
v0.0.161
v0.0.162
v0.0.163
v0.0.164
v0.0.165
v0.0.166
v0.0.167
v0.0.168
v0.0.169
v0.0.170
v0.0.171
v0.0.172
v0.0.173
v0.0.174
v0.0.175
v0.0.176
v0.0.177
v0.0.178
v0.0.179
v0.0.180
v0.0.181
v0.0.182
v0.0.183
v0.0.184
v0.0.185
v0.0.186
v0.0.187
v0.0.188
v0.0.189
v0.0.190
v0.0.191
v0.0.192
v0.0.193
v0.0.194
v0.0.195
v0.0.196
v0.0.197
v0.0.198
v0.0.199
v0.0.1rc0
v0.0.1rc1
v0.0.1rc2
v0.0.1rc3
v0.0.1rc4
v0.0.2
v0.0.200
v0.0.201
v0.0.202
v0.0.203
v0.0.204
v0.0.205
v0.0.206
v0.0.207
v0.0.208
v0.0.209
v0.0.210
v0.0.211
v0.0.212
v0.0.213
v0.0.214
v0.0.215
v0.0.216
v0.0.217
v0.0.218
v0.0.219
v0.0.220
v0.0.221
v0.0.222
v0.0.223
v0.0.224
v0.0.225
v0.0.226
v0.0.227
v0.0.228
v0.0.229
v0.0.230
v0.0.231
v0.0.232
v0.0.233
v0.0.234
v0.0.235
v0.0.236
v0.0.237
v0.0.238
v0.0.239
v0.0.240
v0.0.240rc0
v0.0.240rc1
v0.0.240rc4
v0.0.242
v0.0.243
v0.0.244
v0.0.245
v0.0.247
v0.0.248
v0.0.249
v0.0.250
v0.0.251
v0.0.252
v0.0.253
v0.0.254
v0.0.255
v0.0.256
v0.0.257
v0.0.258
v0.0.259
v0.0.260
v0.0.261
v0.0.262
v0.0.263
v0.0.264
v0.0.265
v0.0.266
v0.0.267
v0.0.268
v0.0.269
v0.0.270
v0.0.271
v0.0.272
v0.0.273
v0.0.274
v0.0.275
v0.0.276
v0.0.277
v0.0.278
v0.0.279
v0.0.281
v0.0.283
v0.0.284
v0.0.285
v0.0.286
v0.0.287
v0.0.288
v0.0.289
v0.0.290
v0.0.291
v0.0.292
v0.0.293
v0.0.294
v0.0.295
v0.0.296
v0.0.297
v0.0.298
v0.0.299
v0.0.300
v0.0.301
v0.0.302
v0.0.303
v0.0.304
v0.0.305
v0.0.306
v0.0.307
v0.0.308
v0.0.309
v0.0.310
v0.0.311
v0.0.312
v0.0.313
v0.0.314
v0.0.315
v0.0.316
v0.0.317
v0.0.318
v0.0.319
v0.0.320
v0.0.321
v0.0.322
v0.0.323
v0.0.324
v0.0.325
v0.0.326
v0.0.327
v0.0.329
v0.0.330
v0.0.331
v0.0.331rc0
v0.0.331rc1
v0.0.331rc2
v0.0.331rc3
v0.0.332
v0.0.333
v0.0.334
v0.0.335
v0.0.336
v0.0.337
v0.0.338
v0.0.339
v0.0.339rc0
v0.0.339rc1
v0.0.339rc2
v0.0.339rc3
v0.0.340
v0.0.341
v0.0.342
v0.0.343
v0.0.344
v0.0.345
v0.0.346
v0.0.347
v0.0.348
v0.0.349
v0.0.349-rc.1
v0.0.349-rc.2
v0.0.350
v0.0.351
v0.0.352
v0.0.353
v0.0.354
v0.0.4
v0.0.5
v0.0.64
v0.0.65
v0.0.66
v0.0.67
v0.0.68
v0.0.69
v0.0.70
v0.0.71
v0.0.72
v0.0.73
v0.0.74
v0.0.75
v0.0.76
v0.0.77
v0.0.78
v0.0.79
v0.0.80
v0.0.81
v0.0.82
v0.0.83
v0.0.84
v0.0.85
v0.0.86
v0.0.87
v0.0.88
v0.0.89
v0.0.90
v0.0.91
v0.0.92
v0.0.93
v0.0.94
v0.0.95
v0.0.96
v0.0.97
v0.0.98
v0.0.99
v0.1.0
v0.1.1
v0.1.10
v0.1.11
v0.1.12
v0.1.13
v0.1.14
v0.1.15
v0.1.16
v0.1.17rc1
v0.1.2
v0.1.3
v0.1.4
v0.1.5
v0.1.6
v0.1.7
v0.1.8
v0.1.9
${ noResults }
144 Commits (9b3a025f9c806a6f8a00030c7058c689536ae5a0)
Author | SHA1 | Message | Date |
---|---|---|---|
|
677408bfc9
|
core[patch]: fix chat history circular import (#23182) | 3 months ago |
|
2b08e9e265
|
core[patch]: update test to catch circular imports (#23172)
This raises ImportError due to a circular import: ```python from langchain_core import chat_history ``` This does not: ```python from langchain_core import runnables from langchain_core import chat_history ``` Here we update `test_imports` to run each import in a separate subprocess. Open to other ways of doing this! |
3 months ago |
|
e8a8286012
|
core[patch]: runnablewithchathistory from core.runnables (#23136) | 3 months ago |
|
b483bf5095
|
core[minor]: handle boolean data in draw_mermaid (#23135)
This change should address graph rendering issues for edges with boolean data Example from langgraph: ```python from typing import Annotated, TypedDict from langchain_core.messages import AnyMessage from langgraph.graph import END, START, StateGraph from langgraph.graph.message import add_messages class State(TypedDict): messages: Annotated[list[AnyMessage], add_messages] def branch(state: State) -> bool: return 1 + 1 == 3 graph_builder = StateGraph(State) graph_builder.add_node("foo", lambda state: {"messages": [("ai", "foo")]}) graph_builder.add_node("bar", lambda state: {"messages": [("ai", "bar")]}) graph_builder.add_conditional_edges( START, branch, path_map={True: "foo", False: "bar"}, then=END, ) app = graph_builder.compile() print(app.get_graph().draw_mermaid()) ``` Previous behavior: ```python AttributeError: 'bool' object has no attribute 'split' ``` Current behavior: ```python %%{init: {'flowchart': {'curve': 'linear'}}}%% graph TD; __start__[__start__]:::startclass; __end__[__end__]:::endclass; foo([foo]):::otherclass; bar([bar]):::otherclass; __start__ -. ('a',) .-> foo; foo --> __end__; __start__ -. ('b',) .-> bar; bar --> __end__; classDef startclass fill:#ffdfba; classDef endclass fill:#baffc9; classDef otherclass fill:#fad7de; ``` |
3 months ago |
|
bd4b68cd54
|
core: run_in_executor: Wrap StopIteration in RuntimeError (#22997)
- StopIteration can't be set on an asyncio.Future it raises a TypeError and leaves the Future pending forever so we need to convert it to a RuntimeError |
3 months ago |
|
bae82e966a
|
core: In astream_events v2 propagate cancel/break to the inner astream call (#22865)
- previous behavior was for the inner astream to continue running with no interruption - also propagate break in core runnable methods |
3 months ago |
|
035a9c9609
|
core[minor]: Add parent_ids to astream_events API (#22563)
Include a list of parent ids for each event in astream events. |
4 months ago |
|
51005e2776
|
core[minor]: Add an async root listener and with_alisteners method (#22151)
- [x] **Adding AsyncRootListener**: "langchain_core: Adding AsyncRootListener" - **Description:** Adding an AsyncBaseTracer, AsyncRootListener and `with_alistener` function. This is to enable binding async root listener to runnables. This currently only supported for sync listeners. - **Issue:** None - **Dependencies:** None - [x] **Add tests and docs**: Added units tests and example snippet code within the function description of `with_alistener` - [x] **Lint and test**: Run make format_diff, make lint_diff and make test |
4 months ago |
|
9120cf5df2
|
core[patch]: Deduplicate of callback handlers in merge_configs (#22478)
This PR adds deduplication of callback handlers in merge_configs. Fix for this issue: https://github.com/langchain-ai/langchain/issues/22227 The issue appears when the code is: 1) running python >=3.11 2) invokes a runnable from within a runnable 3) binds the callbacks to the child runnable from the parent runnable using with_config In this case, the same callbacks end up appearing twice: (1) the first time from with_config, (2) the second time with langchain automatically propagating them on behalf of the user. Prior to this PR this will emit duplicate events: ```python @tool async def get_items(question: str, callbacks: Callbacks): # <--- Accept callbacks """Ask question""" template = ChatPromptTemplate.from_messages( [ ( "human", "'{question}" ) ] ) chain = template | chat_model.with_config( { "callbacks": callbacks, # <-- Propagate callbacks } ) return await chain.ainvoke({"question": question}) ``` Prior to this PR this will work work correctly (no duplicate events): ```python @tool async def get_items(question: str, callbacks: Callbacks): # <--- Accept callbacks """Ask question""" template = ChatPromptTemplate.from_messages( [ ( "human", "'{question}" ) ] ) chain = template | chat_model return await chain.ainvoke({"question": question}, {"callbacks": callbacks}) ``` This will also work (as long as the user is using python >= 3.11) -- as langchain will automatically propagate callbacks ```python @tool async def get_items(question: str,): """Ask question""" template = ChatPromptTemplate.from_messages( [ ( "human", "'{question}" ) ] ) chain = template | chat_model return await chain.ainvoke({"question": question}) ``` |
4 months ago |
|
58b118544e
|
Use immutable sequence type for batch/batch_as_completed types (#22433)
Thank you for contributing to LangChain! - [ ] **PR title**: "package: description" - Where "package" is whichever of langchain, community, core, experimental, etc. is being modified. Use "docs: ..." for purely docs changes, "templates: ..." for template changes, "infra: ..." for CI changes. - Example: "community: add foobar LLM" - [ ] **PR message**: ***Delete this entire checklist*** and replace with - **Description:** a description of the change - **Issue:** the issue # it fixes, if applicable - **Dependencies:** any dependencies required for this change - **Twitter handle:** if your PR gets announced, and you'd like a mention, we'll gladly shout you out! - [ ] **Add tests and docs**: If you're adding a new integration, please include 1. a test for the integration, preferably unit tests that do not rely on network access, 2. an example notebook showing its use. It lives in `docs/docs/integrations` directory. - [ ] **Lint and test**: Run `make format`, `make lint` and `make test` from the root of the package(s) you've modified. See contribution guidelines for more: https://python.langchain.com/docs/contributing/ Additional guidelines: - Make sure optional dependencies are imported within a function. - Please do not add dependencies to pyproject.toml files (even optional ones) unless they are required for unit tests. - Most PRs should not touch more than one package. - Changes should be backwards compatible. - If you are adding something to community, do not re-import it in langchain. If no one reviews your PR within a few days, please @-mention one of baskaryan, efriis, eyurtsev, ccurme, vbarda, hwchase17. |
4 months ago |
|
ed8e9c437a
|
core: In RunnableSequence pass kwargs to the first step (#22393)
- This is a pattern that shows up occasionally in langgraph questions, people chain a graph to something else after, and want to pass the graph some kwargs (eg. stream_mode) |
4 months ago |
|
ee32369265
|
core[patch]: fix runnable history and add docs (#22283) | 4 months ago |
|
dcec133b85
|
[Core] Update Tracing Interops (#22318)
LangSmith and LangChain context var handling evolved in parallel since originally we didn't expect people to want to interweave the decorator and langchain code. Once we get a new langsmith release, this PR will let you seemlessly hand off between @traceable context and runnable config context so you can arbitrarily nest code. It's expected that this fails right now until we get another release of the SDK |
4 months ago |
|
d61bdeba25
|
core[patch]: allow access RunnableWithFallbacks.runnable attrs (#22139)
RFC, candidate fix for #13095 #22134 |
4 months ago |
|
bbd7015b5d
|
core[patch]: Add `TypeError` handler into `get_graph` of `Runnable` (#19856)
# Description ## Problem `Runnable.get_graph` fails when `InputType` or `OutputType` property raises `TypeError`. - |
4 months ago |
|
50186da0a1
|
infra: rm unused # noqa violations (#22049)
Updating #21137 |
4 months ago |
|
fb6108c8f5
|
core[patch]: In astream_events(version=v2) tap output of root run (#21977)
- if tap_output_iter/aiter is called multiple times for the same run issue events only once - if chat model run is tapped don't issue duplicate on_llm_new_token events - if first chunk arrives after run has ended do not emit it as a stream event --------- Co-authored-by: Eugene Yurtsev <eyurtsev@gmail.com> |
4 months ago |
|
a983465694
|
docs: set default anthropic model (#21988)
`ChatAnthropic()` raises ValidationError. |
4 months ago |
|
b6c8b6f944
|
Fix base.py typo (#21862)
ChatOpenaAI --> ChatOpenAI Thank you for contributing to LangChain! - [ ] **PR title**: "package: description" - Where "package" is whichever of langchain, community, core, experimental, etc. is being modified. Use "docs: ..." for purely docs changes, "templates: ..." for template changes, "infra: ..." for CI changes. - Example: "community: add foobar LLM" - [ ] **PR message**: ***Delete this entire checklist*** and replace with - **Description:** a description of the change - **Issue:** the issue # it fixes, if applicable - **Dependencies:** any dependencies required for this change - **Twitter handle:** if your PR gets announced, and you'd like a mention, we'll gladly shout you out! - [ ] **Add tests and docs**: If you're adding a new integration, please include 1. a test for the integration, preferably unit tests that do not rely on network access, 2. an example notebook showing its use. It lives in `docs/docs/integrations` directory. - [ ] **Lint and test**: Run `make format`, `make lint` and `make test` from the root of the package(s) you've modified. See contribution guidelines for more: https://python.langchain.com/docs/contributing/ Additional guidelines: - Make sure optional dependencies are imported within a function. - Please do not add dependencies to pyproject.toml files (even optional ones) unless they are required for unit tests. - Most PRs should not touch more than one package. - Changes should be backwards compatible. - If you are adding something to community, do not re-import it in langchain. If no one reviews your PR within a few days, please @-mention one of baskaryan, efriis, eyurtsev, hwchase17. |
4 months ago |
|
8b3c5f93f5
|
docs: lcel how to and cheatsheet (#21851) | 4 months ago |
|
b1e7b40b6a
|
core: Tap output of sync iterators for astream_events (#21842)
Thank you for contributing to LangChain! - [ ] **PR title**: "package: description" - Where "package" is whichever of langchain, community, core, experimental, etc. is being modified. Use "docs: ..." for purely docs changes, "templates: ..." for template changes, "infra: ..." for CI changes. - Example: "community: add foobar LLM" - [ ] **PR message**: ***Delete this entire checklist*** and replace with - **Description:** a description of the change - **Issue:** the issue # it fixes, if applicable - **Dependencies:** any dependencies required for this change - **Twitter handle:** if your PR gets announced, and you'd like a mention, we'll gladly shout you out! - [ ] **Add tests and docs**: If you're adding a new integration, please include 1. a test for the integration, preferably unit tests that do not rely on network access, 2. an example notebook showing its use. It lives in `docs/docs/integrations` directory. - [ ] **Lint and test**: Run `make format`, `make lint` and `make test` from the root of the package(s) you've modified. See contribution guidelines for more: https://python.langchain.com/docs/contributing/ Additional guidelines: - Make sure optional dependencies are imported within a function. - Please do not add dependencies to pyproject.toml files (even optional ones) unless they are required for unit tests. - Most PRs should not touch more than one package. - Changes should be backwards compatible. - If you are adding something to community, do not re-import it in langchain. If no one reviews your PR within a few days, please @-mention one of baskaryan, efriis, eyurtsev, hwchase17. |
4 months ago |
|
ca768c8353
|
[Core] Check is async callable (#21714)
To permit proper coercion of objects like the following: ```python class MyAsyncCallable: async def __call__(self, foo): return await ... class MyAsyncGenerator: async def __call__(self, foo): await ... yield ``` |
4 months ago |
|
5c2cfabec6
|
core[minor]: Add v2 implementation of astream events (#21638)
This PR introduces a v2 implementation of astream events that removes intermediate abstractions and fixes some issues with v1 implementation. The v2 implementation significantly reduces relevant code that's associated with the astream events implementation together with overhead. After this PR, the astream events implementation: - Uses an async callback handler - No longer relies on BaseTracer - No longer relies on json patch As a result of this re-write, a number of issues were discovered with the existing implementation. ## Changes in V2 vs. V1 ### on_chat_model_end `output` The outputs associated with `on_chat_model_end` changed depending on whether it was within a chain or not. As a root level runnable the output was: ```python "data": {"output": AIMessageChunk(content="hello world!", id='some id')} ``` As part of a chain the output was: ``` "data": { "output": { "generations": [ [ { "generation_info": None, "message": AIMessageChunk( content="hello world!", id=AnyStr() ), "text": "hello world!", "type": "ChatGenerationChunk", } ] ], "llm_output": None, } }, ``` After this PR, we will always use the simpler representation: ```python "data": {"output": AIMessageChunk(content="hello world!", id='some id')} ``` **NOTE** Non chat models (i.e., regular LLMs) are still associated with the more verbose format. ### Remove some `_stream` events `on_retriever_stream` and `on_tool_stream` events were removed -- these were not real events, but created as an artifact of implementing on top of astream_log. The same information is already available in the `x_on_end` events. ### Propagating Names Names of runnables have been updated to be more consistent ```python model = GenericFakeChatModel(messages=infinite_cycle).configurable_fields( messages=ConfigurableField( id="messages", name="Messages", description="Messages return by the LLM", ) ) ``` Before: ```python "name": "RunnableConfigurableFields", ``` After: ```python "name": "GenericFakeChatModel", ``` ### on_retriever_end on_retriever_end will always return `output` which is a list of documents (rather than a dict containing a key called "documents") ### Retry events Removed the `on_retry` callback handler. It was incorrectly showing that the failed function being retried has invoked `on_chain_end` https://github.com/langchain-ai/langchain/pull/21638/files#diff-e512e3f84daf23029ebcceb11460f1c82056314653673e450a5831147d8cb84dL1394 |
4 months ago |
|
a156aace2b
|
core[patch]:Fix Incorrect listeners parameters for Runnable.with_listeners() and .map() (#20661)
- **Issue:** fix #20509 - @baskaryan, @eyurtsev 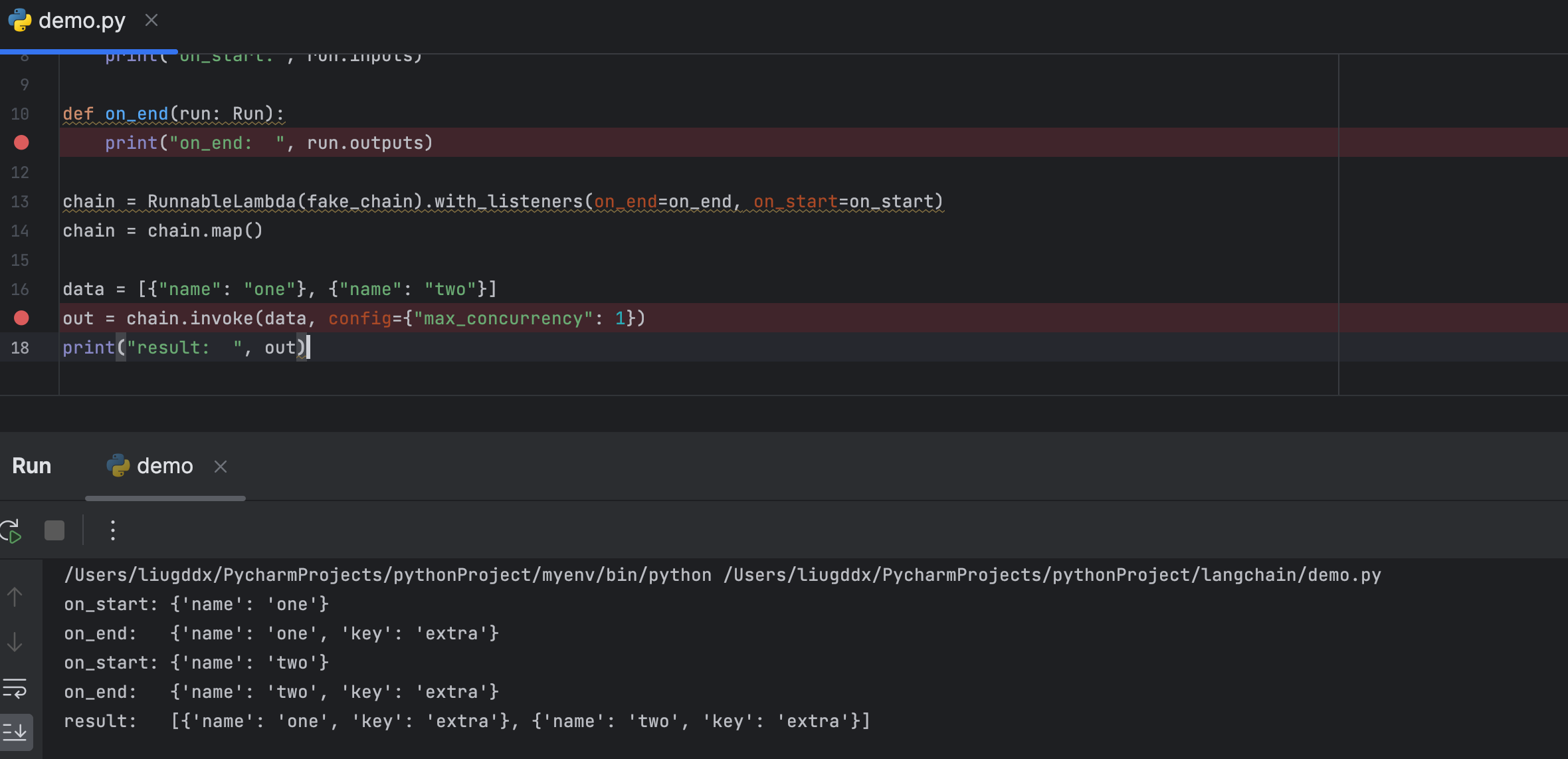 |
4 months ago |
|
ad0f3c14c2
|
core: allow mermaid node labels to have any characters (#21385)
- it's only node ids that are limited Thank you for contributing to LangChain! - [ ] **PR title**: "package: description" - Where "package" is whichever of langchain, community, core, experimental, etc. is being modified. Use "docs: ..." for purely docs changes, "templates: ..." for template changes, "infra: ..." for CI changes. - Example: "community: add foobar LLM" - [ ] **PR message**: ***Delete this entire checklist*** and replace with - **Description:** a description of the change - **Issue:** the issue # it fixes, if applicable - **Dependencies:** any dependencies required for this change - **Twitter handle:** if your PR gets announced, and you'd like a mention, we'll gladly shout you out! - [ ] **Add tests and docs**: If you're adding a new integration, please include 1. a test for the integration, preferably unit tests that do not rely on network access, 2. an example notebook showing its use. It lives in `docs/docs/integrations` directory. - [ ] **Lint and test**: Run `make format`, `make lint` and `make test` from the root of the package(s) you've modified. See contribution guidelines for more: https://python.langchain.com/docs/contributing/ Additional guidelines: - Make sure optional dependencies are imported within a function. - Please do not add dependencies to pyproject.toml files (even optional ones) unless they are required for unit tests. - Most PRs should not touch more than one package. - Changes should be backwards compatible. - If you are adding something to community, do not re-import it in langchain. If no one reviews your PR within a few days, please @-mention one of baskaryan, efriis, eyurtsev, hwchase17. |
5 months ago |
|
6e1e0c7d5c
|
fix: core: draw_mermaid() would create subgroup for edges with same src and tgt (#21275)
Thank you for contributing to LangChain! - [ ] **PR title**: "package: description" - Where "package" is whichever of langchain, community, core, experimental, etc. is being modified. Use "docs: ..." for purely docs changes, "templates: ..." for template changes, "infra: ..." for CI changes. - Example: "community: add foobar LLM" - [ ] **PR message**: ***Delete this entire checklist*** and replace with - **Description:** a description of the change - **Issue:** the issue # it fixes, if applicable - **Dependencies:** any dependencies required for this change - **Twitter handle:** if your PR gets announced, and you'd like a mention, we'll gladly shout you out! - [ ] **Add tests and docs**: If you're adding a new integration, please include 1. a test for the integration, preferably unit tests that do not rely on network access, 2. an example notebook showing its use. It lives in `docs/docs/integrations` directory. - [ ] **Lint and test**: Run `make format`, `make lint` and `make test` from the root of the package(s) you've modified. See contribution guidelines for more: https://python.langchain.com/docs/contributing/ Additional guidelines: - Make sure optional dependencies are imported within a function. - Please do not add dependencies to pyproject.toml files (even optional ones) unless they are required for unit tests. - Most PRs should not touch more than one package. - Changes should be backwards compatible. - If you are adding something to community, do not re-import it in langchain. If no one reviews your PR within a few days, please @-mention one of baskaryan, efriis, eyurtsev, hwchase17. |
5 months ago |
|
1a2ff56cd8
|
core[patch[: docstring update (#21036)
Added missed docstrings. Updated docstrings to consistent format. |
5 months ago |
|
9fa9f05e5d
|
Catch System Error in ast parse (#20961)
I can't seem to reproduce, but i got this: ``` SystemError: AST constructor recursion depth mismatch (before=102, after=37) ``` And the operation isn't critical for the actual forward pass so seems preferable to expand our caught exceptions |
5 months ago |
|
43c041cda5
|
support messages in messages out (#20862) | 5 months ago |
|
477eb1745c
|
Better support for subgraphs in graph viz (#20840) | 5 months ago |
|
a2cc9b55ba
|
core[patch]: Remove autoupgrade to addable dict in Runnable/RunnableLambda/RunnablePassthrough transform (#20677)
Causes an issue for this code ```python from langchain.chat_models.openai import ChatOpenAI from langchain.output_parsers.openai_tools import JsonOutputToolsParser from langchain.schema import SystemMessage prompt = SystemMessage(content="You are a nice assistant.") + "{question}" llm = ChatOpenAI( model_kwargs={ "tools": [ { "type": "function", "function": { "name": "web_search", "description": "Searches the web for the answer to the question.", "parameters": { "type": "object", "properties": { "query": { "type": "string", "description": "The question to search for.", }, }, }, }, } ], }, streaming=True, ) parser = JsonOutputToolsParser(first_tool_only=True) llm_chain = prompt | llm | parser | (lambda x: x) for chunk in llm_chain.stream({"question": "tell me more about turtles"}): print(chunk) # message = llm_chain.invoke({"question": "tell me more about turtles"}) # print(message) ``` Instead by definition, we'll assume that RunnableLambdas consume the entire stream and that if the stream isn't addable then it's the last message of the stream that's in the usable format. --- If users want to use addable dicts, they can wrap the dict in an AddableDict class. --- Likely, need to follow up with the same change for other places in the code that do the upgrade |
5 months ago |
|
48307e46a3
|
core[patch]: Fix runnable map ser/de (#20631) | 5 months ago |
|
719da8746e
|
core: fix attributeerror in runnablelambda.deps (#20569)
- would happen when user's code tries to access attritbute that doesnt exist, we prefer to let this crash in the user's code, rather than here - also catch more cases where a runnable is invoked/streamed inside a lambda. before we weren't seeing these as deps |
5 months ago |
|
806a54908c
|
Runnable graph viz improvements (#20529)
- Add conditional: bool property to json representation of the graphs - Add option to generate mermaid graph stripped of styles (useful as a text representation of graph) |
5 months ago |
|
f3aa26d6bf
|
Fix getattr in runnable binding for cases where config is passed in as arg too (#20528)
…s arg too Thank you for contributing to LangChain! - [ ] **PR title**: "package: description" - Where "package" is whichever of langchain, community, core, experimental, etc. is being modified. Use "docs: ..." for purely docs changes, "templates: ..." for template changes, "infra: ..." for CI changes. - Example: "community: add foobar LLM" - [ ] **PR message**: ***Delete this entire checklist*** and replace with - **Description:** a description of the change - **Issue:** the issue # it fixes, if applicable - **Dependencies:** any dependencies required for this change - **Twitter handle:** if your PR gets announced, and you'd like a mention, we'll gladly shout you out! - [ ] **Add tests and docs**: If you're adding a new integration, please include 1. a test for the integration, preferably unit tests that do not rely on network access, 2. an example notebook showing its use. It lives in `docs/docs/integrations` directory. - [ ] **Lint and test**: Run `make format`, `make lint` and `make test` from the root of the package(s) you've modified. See contribution guidelines for more: https://python.langchain.com/docs/contributing/ Additional guidelines: - Make sure optional dependencies are imported within a function. - Please do not add dependencies to pyproject.toml files (even optional ones) unless they are required for unit tests. - Most PRs should not touch more than one package. - Changes should be backwards compatible. - If you are adding something to community, do not re-import it in langchain. If no one reviews your PR within a few days, please @-mention one of baskaryan, efriis, eyurtsev, hwchase17. |
5 months ago |
|
7997f3b7f8
|
core: forward config params to default (#20402)
nuno's fault not mine --------- Co-authored-by: Nuno Campos <nuno@boringbits.io> Co-authored-by: Nuno Campos <nuno@langchain.dev> |
5 months ago |
|
97b2191e99
|
core: Add concept of conditional edge to graph rendering (#20480)
- implement for mermaid, graphviz and ascii - this is to be used in langgraph |
5 months ago |
|
60c7a17781
|
Remove logic to exclude intermediate nodes from rendering time (#20459)
Description: For simplicity, migrate the logic of excluding intermediate nodes in the .get_graph() of langgraph package (https://github.com/langchain-ai/langgraph/pull/310) at graph creation time instead of graph rendering time. Note: #20381 needs to be approved first --------- Co-authored-by: Angel Igareta <angel.igareta@klarna.com> Co-authored-by: Nuno Campos <nuno@langchain.dev> Co-authored-by: Nuno Campos <nuno@boringbits.io> |
5 months ago |
|
d55a365c6c
|
Fix CDN URL in mermaid graph renderer (#20381)
Description of features on mermaid graph renderer: - Fixing CDN to use official Mermaid JS CDN: https://www.jsdelivr.com/package/npm/mermaid?tab=files - Add device_scale_factor to allow increasing quality of resulting PNG. |
5 months ago |
|
ece008f117
|
docs: Refine RunnablePassthrough docstring (#19812)
Description: This update refines the documentation for `RunnablePassthrough` by removing an unnecessary import and correcting a minor syntactical error in the example provided. This change enhances the clarity and correctness of the documentation, ensuring that users have a more accurate guide to follow. Issue: N/A Dependencies: None This PR focuses solely on documentation improvements, specifically targeting the `RunnablePassthrough` class within the `langchain_core` module. By clarifying the example provided in the docstring, users are offered a more straightforward and error-free guide to utilizing the `RunnablePassthrough` class effectively. As this is a documentation update, it does not include changes that require new integrations, tests, or modifications to dependencies. It adheres to the guidelines of minimal package interference and backward compatibility, ensuring that the overall integrity and functionality of the LangChain package remain unaffected. Thank you for considering this documentation refinement for inclusion in the LangChain project. |
5 months ago |
|
f1248f8d9a
|
core[patch]: configurable init params (#20070)
Proposed fix for #20061. need to test --------- Co-authored-by: Erick Friis <erick@langchain.dev> |
5 months ago |
|
31a641a155
|
core: fix return of draw_mermaid_png and change to not save image by default (#19950)
- **Description:** Improvement for #19599: fixing missing return of graph.draw_mermaid_png and improve it to make the saving of the rendered image optional Co-authored-by: Angel Igareta <angel.igareta@klarna.com> |
6 months ago |
|
2ae6dcdf01
|
core: Assign missing message ids in BaseChatModel (#19863)
- This ensures ids are stable across streamed chunks - Multiple messages in batch call get separate ids - Also fix ids being dropped when combining message chunks Thank you for contributing to LangChain! - [ ] **PR title**: "package: description" - Where "package" is whichever of langchain, community, core, experimental, etc. is being modified. Use "docs: ..." for purely docs changes, "templates: ..." for template changes, "infra: ..." for CI changes. - Example: "community: add foobar LLM" - [ ] **PR message**: ***Delete this entire checklist*** and replace with - **Description:** a description of the change - **Issue:** the issue # it fixes, if applicable - **Dependencies:** any dependencies required for this change - **Twitter handle:** if your PR gets announced, and you'd like a mention, we'll gladly shout you out! - [ ] **Add tests and docs**: If you're adding a new integration, please include 1. a test for the integration, preferably unit tests that do not rely on network access, 2. an example notebook showing its use. It lives in `docs/docs/integrations` directory. - [ ] **Lint and test**: Run `make format`, `make lint` and `make test` from the root of the package(s) you've modified. See contribution guidelines for more: https://python.langchain.com/docs/contributing/ Additional guidelines: - Make sure optional dependencies are imported within a function. - Please do not add dependencies to pyproject.toml files (even optional ones) unless they are required for unit tests. - Most PRs should not touch more than one package. - Changes should be backwards compatible. - If you are adding something to community, do not re-import it in langchain. If no one reviews your PR within a few days, please @-mention one of baskaryan, efriis, eyurtsev, hwchase17. |
6 months ago |
|
d5c412b0a9
|
core: Add docs for RunnableConfigurableFields (#19849)
- [x] **docs**: core: Add docs for `RunnableConfigurableFields` - **Description:** Added incode docs for `RunnableConfigurableFields` with example - **Issue:** #18803 - **Dependencies:** NA - **Twitter handle:** NA --------- Co-authored-by: Chester Curme <chester.curme@gmail.com> |
6 months ago |
|
c2ccf22dfd
|
core: generate mermaid syntax and render visual graph (#19599)
- **Description:** Add functionality to generate Mermaid syntax and render flowcharts from graph data. This includes support for custom node colors and edge curve styles, as well as the ability to export the generated graphs to PNG images using either the Mermaid.INK API or Pyppeteer for local rendering. - **Dependencies:** Optional dependencies are `pyppeteer` if rendering wants to be done using Pypeteer and Javascript code. --------- Co-authored-by: Angel Igareta <angel.igareta@klarna.com> Co-authored-by: Bagatur <22008038+baskaryan@users.noreply.github.com> |
6 months ago |
|
263ee78886
|
core[runnables]: docstring for class RunnableSerializable, method configurable_fields (#19722)
**Description:** Update to the docstring for class RunnableSerializable, method configurable_fields **Issue:** [Add in code documentation to core Runnable methods #18804](https://github.com/langchain-ai/langchain/issues/18804) **Dependencies:** None --------- Co-authored-by: Chester Curme <chester.curme@gmail.com> |
6 months ago |
|
ec4dcfca7f
|
core[runnables]: docstring of class RunnableSerializable, method configurable_alternatives (#19724)
**Description:** Update to the docstring for class RunnableSerializable, method configurable_alternatives **Issue:** [Add in code documentation to core Runnable methods #18804](https://github.com/langchain-ai/langchain/issues/18804) **Dependencies:** None --------- Co-authored-by: Chester Curme <chester.curme@gmail.com> |
6 months ago |
|
bcb8ab5216
|
docs: Improve docstring for Runnable bind method (#19659)
Added example to the docstring of the "bind" method of Runnable. This makes it easier to understand the purpose of the method when reviewing in code editors. E.g. VS Code below. <img width="833" alt="Screenshot 2024-03-27 at 16 24 18" src="https://github.com/langchain-ai/langchain/assets/45722942/ad022d4e-7bc0-4f4b-aa7a-838f1816cc52"> --------- Co-authored-by: Chester Curme <chester.curme@gmail.com> |
6 months ago |
|
1f422318b7
|
core[minor]: Use BaseChatMessageHistory async methods in RunnableWithMessageHistory (#19565)
Co-authored-by: Eugene Yurtsev <eyurtsev@gmail.com> |
6 months ago |
|
c93d4ea91c
|
docs: Add in code documentation to core Runnable map methods (docs only) (#19517)
- **Issue:** #18804 - @baskaryan, @eyurtsev |
6 months ago |